WPF FlowDocuments can contain controls like CheckBoxes and Buttons. When the document is displayed statically and is not editable the controls are enabled, however in WPF V1 when they are rendered as the content of a RichTextBox (and the FlowDocument can be edited) the controls are disabled.
This has not always been the case. During the WPF CTP releases around beta 1 it was possible to enable controls inside a FlowDocument contained within a RichTextBox by setting the IsEnabled property on the FlowDocument to true. As WPF V1 approached the WPF team decided to disable this functionality. Although I don’t believe I’ve ever seen a reason for this it would be a difficult feature to fully support.
Fortunately there is a fairly clean work-around, for those prepared to take the risks associated with using a feature that the product team has consciously disabled. By inheriting from FlowDocument and over-riding the protected IsEnabledCore method to return true, and then using this FlowDocument sub-type inside the RichTextBox you can add enabled controls.
C# Code (WPF V1)class EnabledFlowDocument : FlowDocument
{
protected override bool IsEnabledCore
{
get
{
return true;
}
}
}
Xaml Code (WPF V1)
<RichTextBox Grid.Column=“1”>
<!– sub-class of FlowDocument –>
<my:EnabledFlowDocument FontFamily=“Segoe” FontSize=“12”>
<Paragraph>
This is some text inside a sub-type of flowdocument
</Paragraph>
<BlockUIContainer>
<Button Content=“Click Me!” Click=“ButtonClicked”>
</Button>
</BlockUIContainer>
<Paragraph>
<Hyperlink
NavigateUri=“http://learnwpf.com" Click=“LinkClicked”>
visit LearnWPF.com
</Hyperlink>
</Paragraph>
</my:EnabledFlowDocument>
</RichTextBox>
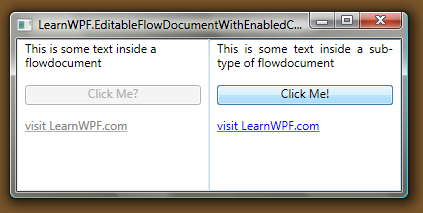
You can download the source code here
Note: I had to explicitly set the FontFamily and FontSize on the instance of the sub-type of FlowDocument that I created to match the “default” appearance of the regular FlowDocument, so a little more work is required to make this a drop-in replacement for the regular FlowDocument.
I first saw this work-around in the MSDN forums but have subsequently discovered it has been documented elsewhere.
Comments
Controls in InlineUIContainer & BlockUIContainer always disabled in RichTextBox.Document | Jptab
Controls in InlineUIContainer & BlockUIContainer always disabled in RichTextBox.Document - Programmers Goodies