The WPF layout system is very, very good. I find it infinitely better than CSS for layout, but occasionally (very occasionally) things don’t quite work out the way you want which is where the list of tips and tricks comes in.
Grid (and maybe StackPanel)
First off let me offer a suggestion. For all things layout, start with the Grid. Except for the WrapPanel (and other custom panels you may have made) the Grid is the ‘super-set’ of layout panels. It is no accident that the default root layout container that Expression Blend and Visual Studio add to every Window and UserControl is the Grid. Use auto-sized rows and columns for things that you want to only take up as much room as they need. Use star (*) sizing for things you want to be as big as possible. Use column and row spanning to reduce the number of grids you need to use, and the need for nested grids. Add grid splitters to allow the users to re-size parts of the grid. If you want to have multiple grids that share column widths look at shared size groups.
Although there certainly are some cases where I use other types of panels the grid is an excellent default, followed (distantly) by the StackPanel which can be good for very simple layouts. WrapPanel has a very specific niche use. I’d treat the use of other panels with some circumspection.
Snoop is your friend
As with almost all things WPF Snoop is your friend and an indispensible utility for looking in to layout problems. The first great thing about snoop is that you can interactively change the layout properties of elements in a running application and observe what effect it has on the layout. The can be very useful if it takes a number of steps to get your UI into the state where it is having the problem, because you can investigate the effects of changing a number of different properties without re-compiling your application.
When investigating layout issues with snoop one technique is to simply walk the visual tree of elements looking for elements with un-usual or unexpected layout properties. There are many properties that effect layout, and until recently no way in snoop to select a group of properties to view, however this has been addressed in recent builds. By dropping down the combo box on the top-right of the screen (with the red rectangle shown below) we can look at just the layout-related properties. Snoop allows you to define custom lists of properties you want to see (by selecting the ‘Edit Filters’ option at the bottom of the list). I usually do this and add properties like VerticalAlignment, VerticalContentAlignment etc. to the list when debugging layout issues, as these properties can also effect layout.
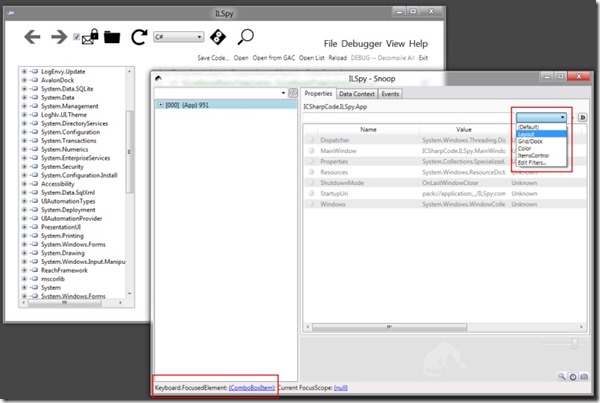
Snoop also allows you to hold down CTRL+SHIFT and mouse over elements of interest (selecting them in the tree-view on the left and allowing you to view their properties) or click on the link at the bottom of the window in snoop to select the item that currently has keyboard focus in the tree.
Simplify
The more complex the layout the more difficult it will be to troubleshoot layout problems. Often an inability to bend a layout to your will can be a sign that things have become too complicated, and a good first step in fixing a layout problem can be to look to dramatically simplify. Fortunately Blend and Visual Studio often leave lots of low-hanging fruit – you should be looking to remove redundant nested panels, such as grids nested immediately inside other panels, panels with only 1 child, and redundant attributes. Also be on the look-out for redundant transforms. Use VerticalAlignment and HorizontalAlignment where appropriate to avoid complicated margins and nested elements.
Avoid fixed sizes for things
The root cause of many layout problems is fixed sizes being applied (or rather mis-applied) to elements, with unintended consequences on the element (when the content changes) or around it. Many of these kinds of problems can be avoided altogether by just not setting absolute sizes on things. Absolute values are completely appropriate for margins and padding values, but you should treat absolute size values for content elements with suspicion. Instead a combination of correct use of the Grid, consideration of vertical and horizontal alignments, and appropriate margins and padding can usually achieve anything that is required. One exception to this guideline is images that are compiled in to the application – the sizes of these are known in advance, and can be set in the XAML or code.
Build a Prototype
I know it sounds trite and obvious, but one of the best things to do is fire up Kaxaml and create the simplest version of what you’re trying to create. Does that work? If not, why not?
»