The query-string portion of the URL in a HTTP request is a useful place for passing state between pages in web applications. It is therefore not unexpected that developers would also want to use this common idiom for passing information to WPF XBAPs (Xaml Browser Applications) that they wish to integrate with their site.
Fortunately this is not too difficult, except there is one trick you need to know. The ActivationUri property of the ApplicationDeployment object gives you the Uri that launched the XBAP. To get access to this you need to add a reference to System.Deployment.dll. To get hold of the current ApplicationDeployment object for your XBAP use the static CurrentDeployment property of the ApplicationDeployment object like so.
Uri launchUri = System.Deployment.Application.ApplicationDeployment.CurrentDeployment.ActivationUri;
If you launch this code from inside Visual Studio to debug it won’t work. The way it is launched means when you press F5 means that the CurrentDeployment property will be null and thus cause a NullReferenceException. You can use the static IsNetworkDeployed property of the ApplicationDeployment ojbect to check if you’ve been deployed over the network (for an XBAP application hosted in the browser this should be true).
if (ApplicationDeployment.IsNetworkDeployed)
{
Uri launchUri = ApplicationDeployment.CurrentDeployment.ActivationUri;
}
So far so good, and now for the trick. In order for the deployment infrastructure to actually pass anything through to you via this API you need to set a property in your manifest. Fortunately Visual Studio 2005 gives you a convenient way to do this. Select project properties in Visual Studio 2005 and then go to the publish tab, as shown in figure below.
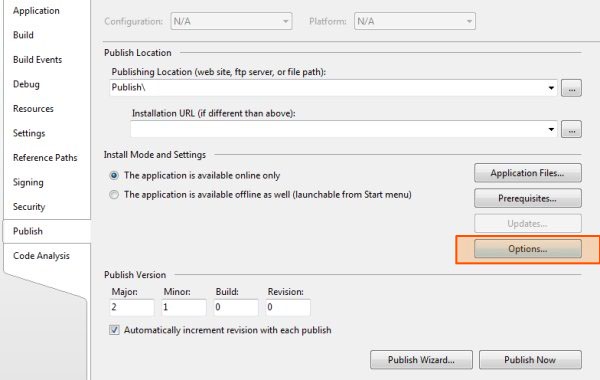
Now click the Options button and in the options dialog check the box that says “Allow URL parameters to be passed to application”. Click OK and re-build your application.
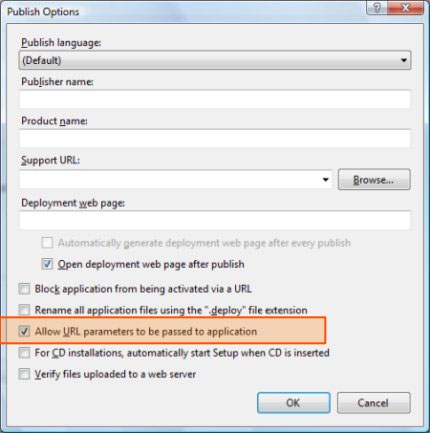
Once you’ve verified that the URI is being passed through you can use the Query property of the URI to return the query-string portion, and parse out any information you need from there.
»