Humans live in an analogue world, where even the most uniform-looking things display some variation in color. Subtle gradients are one type of color variation typically seen on ‘real-world’ objects, and are well understood in software UIs (to the point of abuse). Real-world objects also often exhibit variations in color caused by the texture (sometimes these are quite striking). When we see an image like the one below we immediately know how this particular object would feel to touch, how soft it would be and maybe even how it would smell.
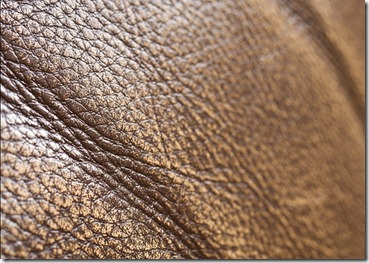
In contrast with all this things in the digital world are often much flatter and less visually interesting, without any of the suggestion of texture and the associations they bring. Complex textures (like leather) are usually best achieved with photos of real-world objects, but increasingly subtle use of digital noise is being used to create a little bit of visual variation to otherwise flat UIs.
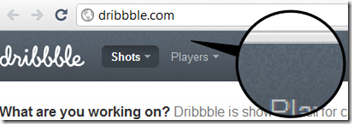
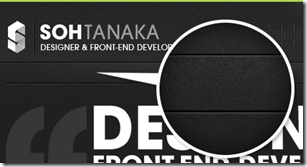
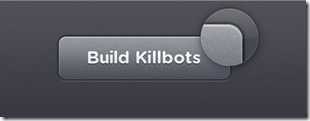
So how can I use this in my WPF application?
The simplest way to add noise to your WPF applications has been to create a ‘noisy’ texture using an imaging program like Photoshop, the GIMP or Paint.NET, and use it as an image source to an ImageBrush in WPF. If you’re working with a visual designer this is probably what they’ll do. One pretty common trick here is to make the noise image almost completely transparent – it can then be layered over the top of gradients and the like to create more complicated visual effects which would otherwise need to be created as a single large bitmap. This technique can be seen in ‘SimpleNoise’ sample application in the download. An ImageBrush is defined like so, using a ‘noise’ PNG image that has been created with the GIMP added as a Resource to the project as the ImageSource:
<ImageBrush x:Key="noise" ImageSource="noise.png" TileMode="Tile" Viewport="0,0 128,128" ViewportUnits="Absolute" />
When overlaid on a dark gradient the following dark and slightly noisy background is created. In the sample shown a small amount of noise has also been added to background of the light block of text in the middle.
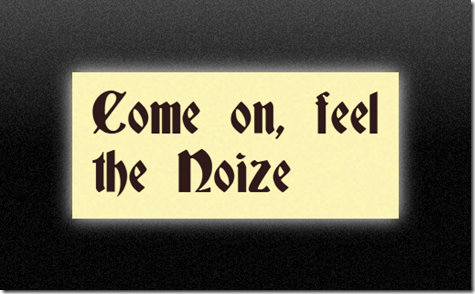
Programmers do it in code
If you’re a developer your Photoshop muscles may be weak or non-existent. Also the ‘static’ approach has a number of drawbacks. Textures that look good over dark backgrounds will not work well with light ones, or textures created for one color may not suit another. Each texture you add increases the size of your application, and if your application needs to support a number of themes or dynamic color schemes it can become a bit of a pain to generate multiple ‘noise’ image. For these reasons I created a programmatic means of creating noisy textures in a WPF application, allowing the developer to specify the different colors to be used, and the frequency with which they should appear. This can be seen in the ‘ComeOnFeelTheNoize’ project in the download. Once again an ImageBrush is used over the top of an object with a dark gradient as the background. This time however the ImageSource for the brush is bound to another resource called NoiseGenerator:
<n:NoiseGenerator x:Key="background_noise" Size="128">
<n:NoiseGenerator.Colors>
<n:ColorFrequency Color="#33222222" Frequency="30" />
<n:ColorFrequency Color="#3300A500" Frequency="1" />
<n:ColorFrequency Color="#55E0E0E0" Frequency="15" />
<n:ColorFrequency Color="#33666666" Frequency="15" />
</n:NoiseGenerator.Colors>
</n:NoiseGenerator>
<ImageBrush ImageSource="{Binding Source={StaticResource background_noise}, Path=NoiseImage}" x:Key="noise" TileMode="Tile" ViewportUnits="Absolute" Viewport="0,0 128,128" />
Once again, when the noisy ImageBrush is overlaid on a dark gradient a very similar dark and slightly noisy background is created. The NoiseGenerator has also been used to create a noisy background for the light block of text in the middle.
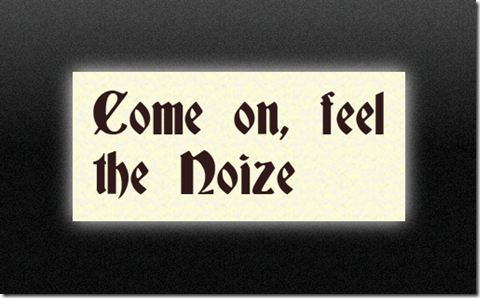
The NoiseGenerator uses a very simplistic algorithm to generate the noise image. One enhancement would be to implement alternate ‘noise’ algorithms which can suggest materials of a different ‘’texture’ to the eye.
Alternative Approaches
Aside from the super-simple approach of creating an ‘noise’ image file using an image editing tool there are a few other approaches to getting programmatic noise. One alternative approach would be to add noise via a pixel-shader.
So finally, everyone..this time with feeling! Come on, feel the noize.
Leather image courtesy of smig44_uk
»